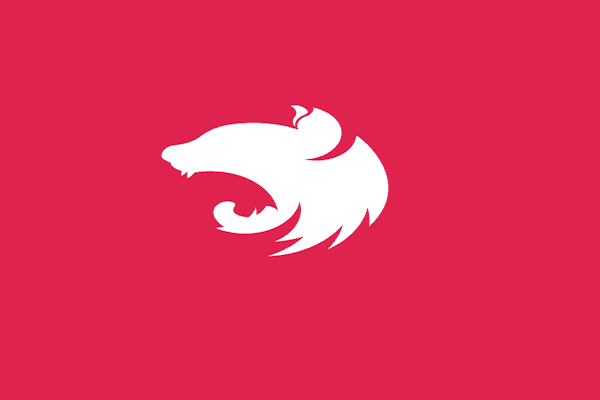
Project Files
Prisma Schema
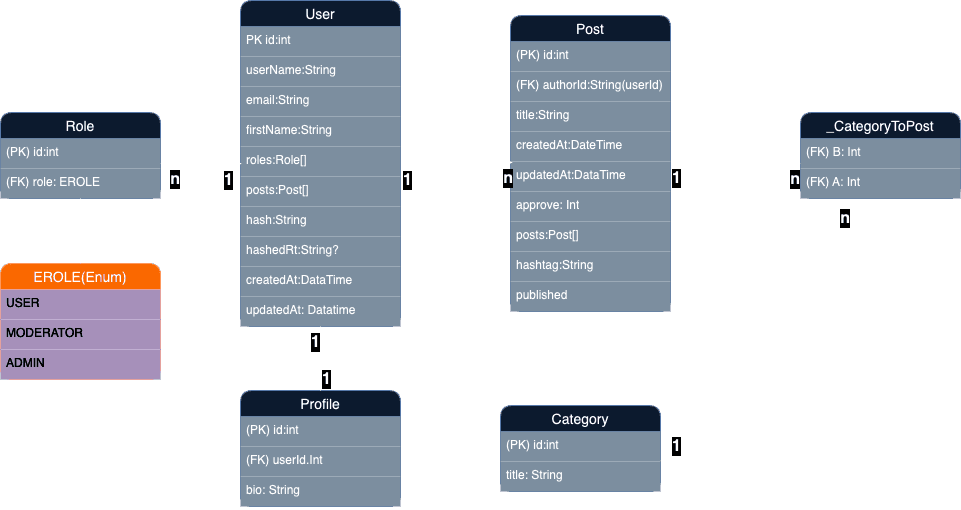
About the project
- User can register and login Brearer Token
- User can register and login through Brearer Token
- Author can register.
- The author can write an article or edit her own article.
- Unregistered users cannot edit.
- Admin cannot access authors' articles directly
- Safe exit
The project is coded with nestjs, which is the node.js Framework. Nestjs typescript was used
🔨 Run the App
npm install
Running the app
Docker
- Install
Docker Desktop
- Open Terminal under resources folder to run
PostgreSQL
andPgAdmin
on Docker Container
development
docker-compose up -d
Nestjs
-
Installation
npm install
-
Run
//development npm run start // watch mode npm run start:dev // production mode npm run start:prod
Test
Swagger Screenshot
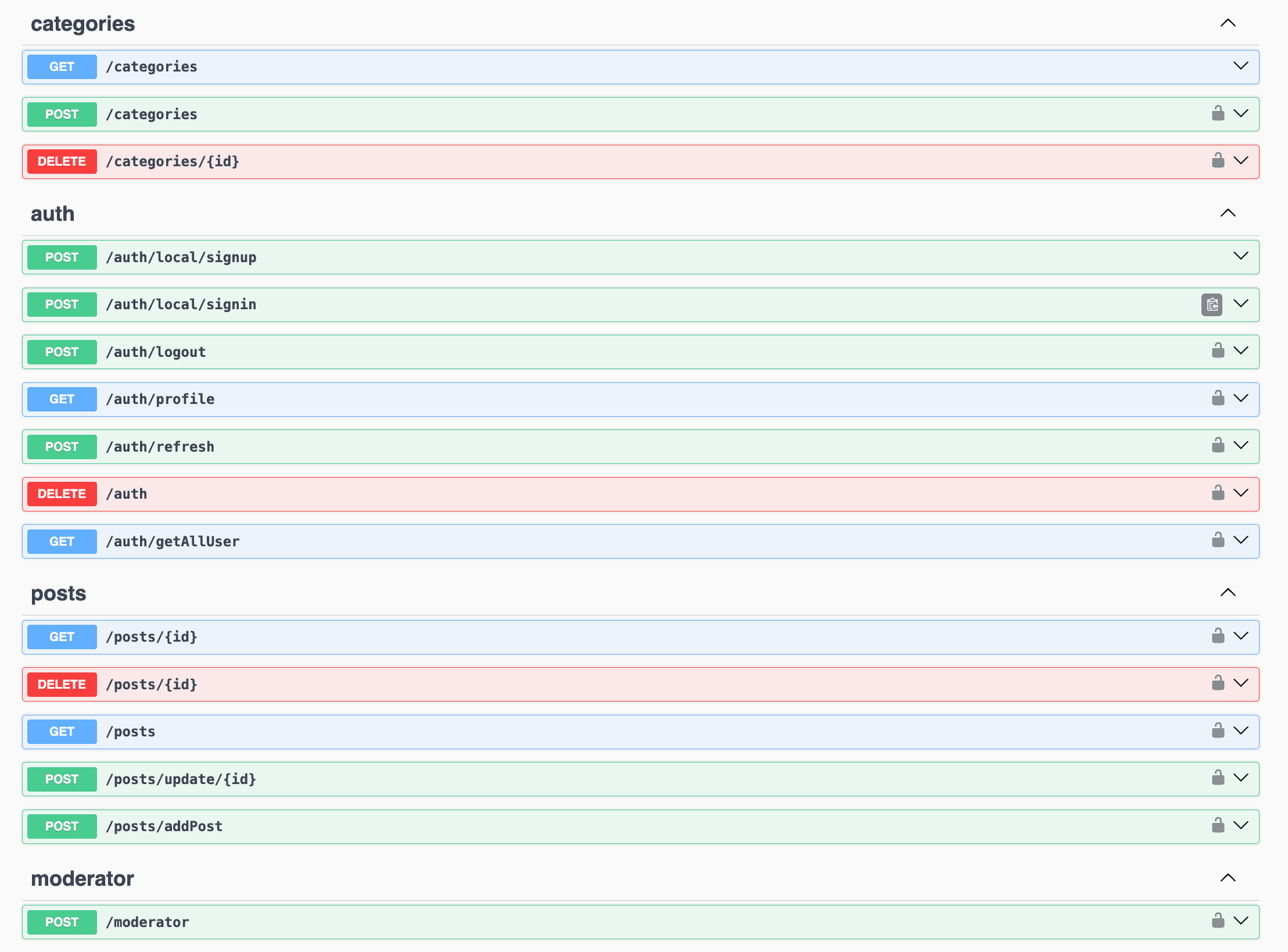
http://localhost:3333/api
Explore Rest APIs
Method | Url | Description |
---|---|---|
POST | auth/local/signup | Sign Up for User , Admin and Moderator |
POST | auth/local/signin | Sign Up for User , Admin and Moderator |
POST | auth/logout | Logout |
GET | auth/profile/(id) | Displays user profiles |
POST | auth/refresh | Gets refresh-token and generates access-token |
DELETE | auth | Delete of User |
GET | auth/getAllUser | Get all advertisements From Admin of User |
GET | posts/(id) | Post by id is all users or client |
DELETE | posts/(id) | Post MODERATOR deletes |
GET | auth | Delete of User |
POST | posts/update/(id) | Get post by Id From MODERATOR |
POST | posts/addPost | Get post by Id From MODERATOR |
GET | categories | Get all client |
POST | categories | Create category |
DELETE | categories/(id) | Delete category by id |
POST | moderator | Careate Author is user and profile |
Valid Request Body
Sign Up for User, Admin and Moderator
// http://localhost:3333/auth/local/signup
{
"email": "sefa.demirtas91@gmail.com",
"password": "test",
"roles": [
"ADMIN",
"MODERATOR",
"USER"
]
}
Login
// http://localhost:3333/auth/local/login
// Request
Bearer Token : Access Token of User
{
"email": "sefa.demirtas91@gmail.com",
"password": "string",
}
// Response
{
"access_token":
"eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJzdWIiOjEsInJvbGVzIjpbeyJpZCI6MSwicm9sZSI6IlVTRVIiLCJ1c2VySWQiOjF9XSwiZW1haWwiOiJzZWZhLmRlbWlydGFzOTFAZ21haWwuY29tIiwiaWF0IjoxNjk4NzQ1NzY2LCJleHAiOjE2OTg3NDY2NjZ9.4Mtzi7SdLOqLVX5O911fbtKOIt9akuGmmzlhA_34GAo",
"refresh_token": "eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJzdWIiOjEsInJvbGVzIjpbeyJpZCI6MSwicm9sZSI6IlVTRVIiLCJ1c2VySWQiOjF9XSwiZW1haWwiOiJzZWZhLmRlbWlydGFzOTFAZ21haWwuY29tIiwiaWF0IjoxNjk4NzQ1NzY2LCJleHAiOjE2OTkzNTA1NjZ9.JzBJ64ykxlXi_uqIGiRKcjgUJd4ZJemi9V9W8pPs5RY"
}
Sign Up for User, Admin and Moderator
// http://localhost:3333/posts/addPost
{
"title": "string",
"published": true,
"authorId": 0,
"approve": 0,
"hashtag": "string",
"story": "string",
"categories": [
"string"
]
}
Create category of ADMIN
// http://localhost:3333/categories
{
"name": "string"
}
// Bearer Token : Access Token of ADMIN @GetCurrentUserId() decorator id anr @Role decorator is role ADMIN
Careate Author is user and profileN
// http://localhost:3333/moderator
{
"updatedAt": "2023-10-31T11:38:16.182Z",
"userName": "string",
"email": "string",
"firstName": "string",
"lastName": "string",
"profileBio": "string"
}
// Bearer Token : Access Token of MODERATOR @GetCurrentUserId() decorator id and @Role decorator is role MODERATOR
Valid Request Params
Get post by Id From all User(Public)
http://localhost:3333/posts/{id}
Profile
http://localhost:3333/auth/profile/{id}
All client or client
Delete by id
http://localhost:3333/auth/profile/(id)
The moderator is a Autor.@Role(MODERATOR)
Profile
http://localhost:3333/categories/(id)
Bearer Token : Access Token of ADMIN @@GetCurrentUserId() decorator id anr @Role decorator is role ADMIN
Valid Request Params and Body
Get post by Id From MODERATOR
// http://localhost:3333/posts/update/(id)
{
"updatedAt": "2023-10-31T11:07:05.591Z",
"title": "string",
"published": true,
"authorId": 0,
"approve": 0,
"hashtag": "string",
"story": "string"
}
// Bearer Token : Access Token of MODERATOR @Role(MODERATOR)
No Request or Params
Logout
http://localhost:3333/auth/logout
Bearer Token : Access Token of Admin , User or Moderator
Refresh
http://localhost:3333/auth/refresh
Bearer Token : Refresh Token of Admin , User or Moderator
User Delete
http://localhost:3333/auth/auth
Bearer Token : Access Token of Admin , User , Admin or Moderator @@GetCurrentUserId() decorator id
all advertisements From Admin of User
http://localhost:3333/auth/getAllUser
Bearer Token : Access Token of ADMIN @@GetCurrentUserId() decorator id anr @Role decorator is role ADMIN
Get all posts is client or user
http://localhost:3333/posts
All user or all clienr
Get all posts is client or user(Public)
http://localhost:3333/categories
All user or all client
Support
Projeck Blog is an MIT-licensed open source project.
License
Project is MIT licensed.
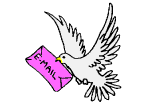
Table Of Content
- Project Files
- Prisma Schema
- About the project
- 🔨 Run the App
- Running the app
- Docker
- Nestjs
- Test
- Valid Request Body
- Sign Up for User, Admin and Moderator
- Login
- Sign Up for User, Admin and Moderator
- Create category of ADMIN
- Careate Author is user and profileN
- Valid Request Params
- Get post by Id From all User(Public)
- Profile
- Delete by id
- Profile
- Valid Request Params and Body
- Get post by Id From MODERATOR
- No Request or Params
- Logout
- Refresh
- User Delete
- all advertisements From Admin of User
- Get all posts is client or user
- Get all posts is client or user(Public)
- Support
- License